I was looking for a way to generate a deterministic set of random permutations every day. Nowadays, I usually give ChatGPT a try first but didn’t get the answer I want on this one.
I ran into this need while making this webpage to show some generated coloring pages in a waterfall layout.
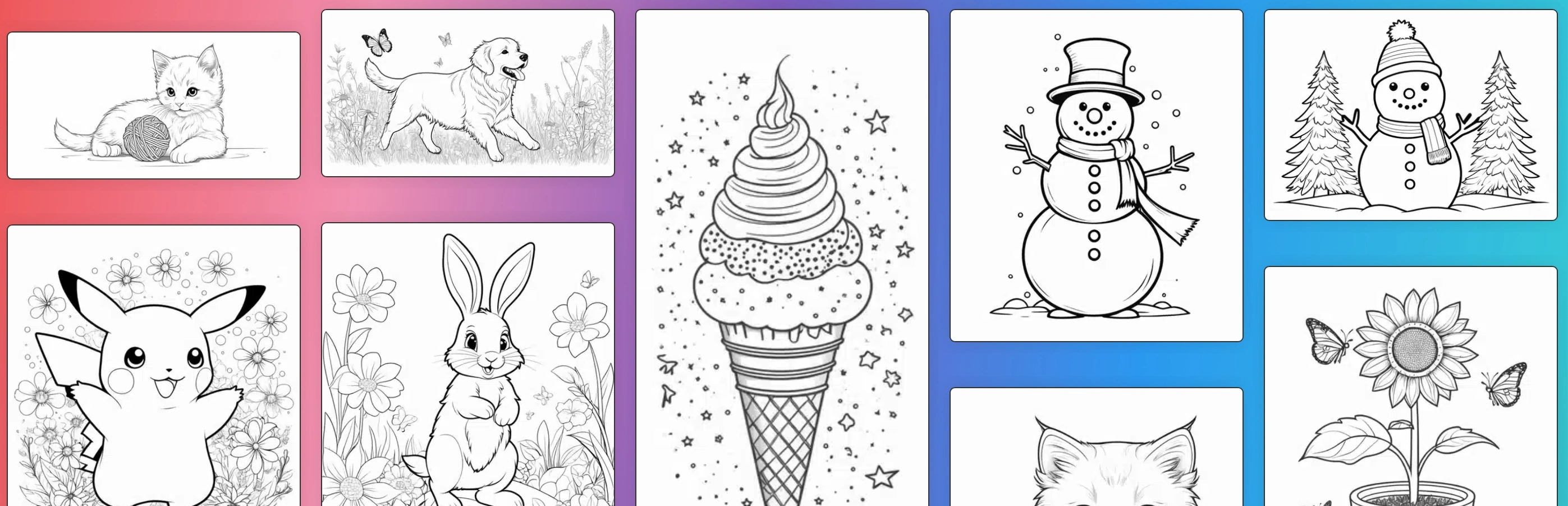
To elaborate on the request, this is meant to give the user a different landing page view each time they come to this website. But I don’t want it to be totally randomized; on the same day, if a user remembers the location of a certain picture, there should be a way the user can find it. The next time when the user gets a different landing page unexpectedly, I assume the most intuitive reaction is to refresh the page a couple of times to hope to get it back.
In order to achieve this behavior, I will need to randomize the indices of images each time the user refreshes the page. But the total number of patterns should be limited. In the following code snippet, the total number of patterns is 4. Furthermore, I want this set of 4 patterns to change day over day so that each day, the 4 patterns are different.
var currentTimestamp = new Date().getTime(); var daySeed = (Math.floor(currentTimestamp/1000/60/60/24) % 1000) / 1000 * Math.PI; var randomPattern = Math.floor(currentTimestamp)%4; for (let i = inds.length-1; i >= 1; i--) { let randVal = Math.sin(randomPattern*daySeed*i)*10000; randVal = (randVal - Math.floor(randVal)); let j = Math.floor(randVal*(i+1)); [inds[j],inds[i]] = [inds[i], inds[j]]; }
It is not very complicated to get this piece of code. We get a daily unique number and construct a random seed from this unique number and 0, 1, 2, 3 respectively, assuming we need 4 patterns. The tricky part is that in JavaScript, there is no way you can set a random seed (according to ChatGPT), so here we have implemented a simple algorithm to randomly shuffle the array.